Lambda Functions in Python and Their Usage
13 February 2025
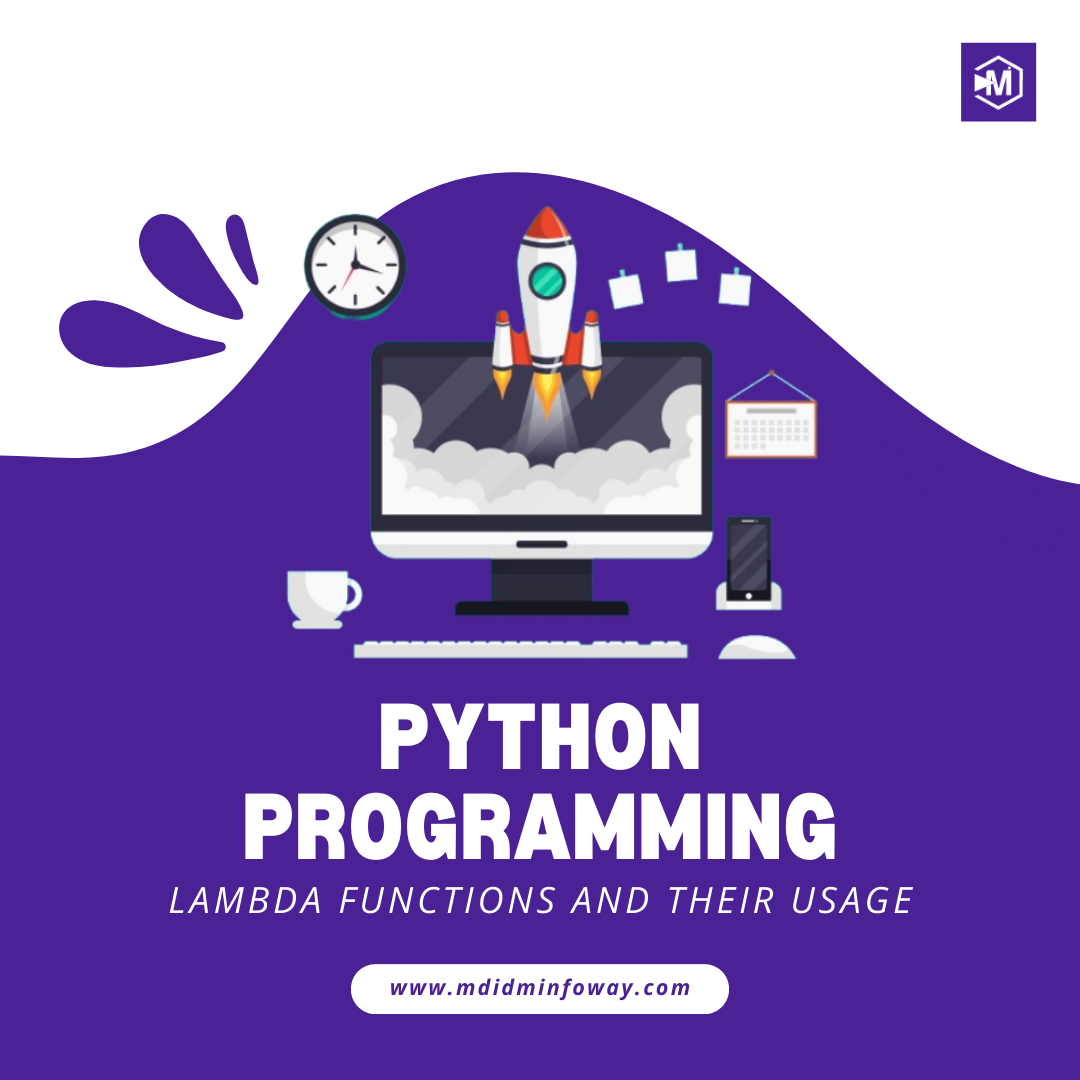
Lambda Functions in Python and Their Usage
Lambda functions, also known as anonymous functions, are small single-expression functions in Python. They are useful for writing concise, inline functions without formally defining them using def.
1. Defining a Lambda Function
A lambda function is defined using the lambda keyword, followed by parameters and an expression.
Syntax:
lambda arguments: expression
lambda - Defines the function.
arguments - The input parameters (can be multiple).
expression - A single expression that is evaluated and returned.
2. Example: Basic Lambda Function
# Regular function
def square(x):
return x ** 2
# Equivalent lambda function
square_lambda = lambda x: x ** 2
# Calling the functions
print(square(5)) # Output: 25
print(square_lambda(5)) # Output: 25
3. Using Lambda Functions in Different Scenarios
a) Using Lambda with map()
The map() function applies a lambda function to each element in an iterable.
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
b) Using Lambda with filter()
The filter() function select elements that satisfy a condition.
numbers = [10, 15, 20, 25, 30]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [10, 20, 30]
c) Using Lambda with sorted()
Lambda functions can define custom sorting criteria.
students = [("Alice", 85), ("Bob", 92), ("Charlie", 78)]
sorted_students = sorted(students, key=lambda x: x[1]) # Sort by scores
print(sorted_students)
# Output: [('Charlie', 78), ('Alice', 85), ('Bob', 92)]
d) Using Lambda in reduce() from functools
The reduce() function applies a rolling computation to elemets in a list.
from functools import reduce
numbers = [1, 2, 3, 4, 5]
sum_result = reduce(lambda x, y: x + y, numbers)
print(sum_result) # Output: 15
4. Key Advantages of Lambda Functions
- Concise - Reduces code length.
- No need for Naming - Useful for quick, throwaway functions.
- Efficient in Functional Programming - Works well with map(), filter(), and reduce().
Lambda functions are best suited for simple operations but should be avoided for complex logic where readability is important.