Instance variables and methods in python
18 February 2025
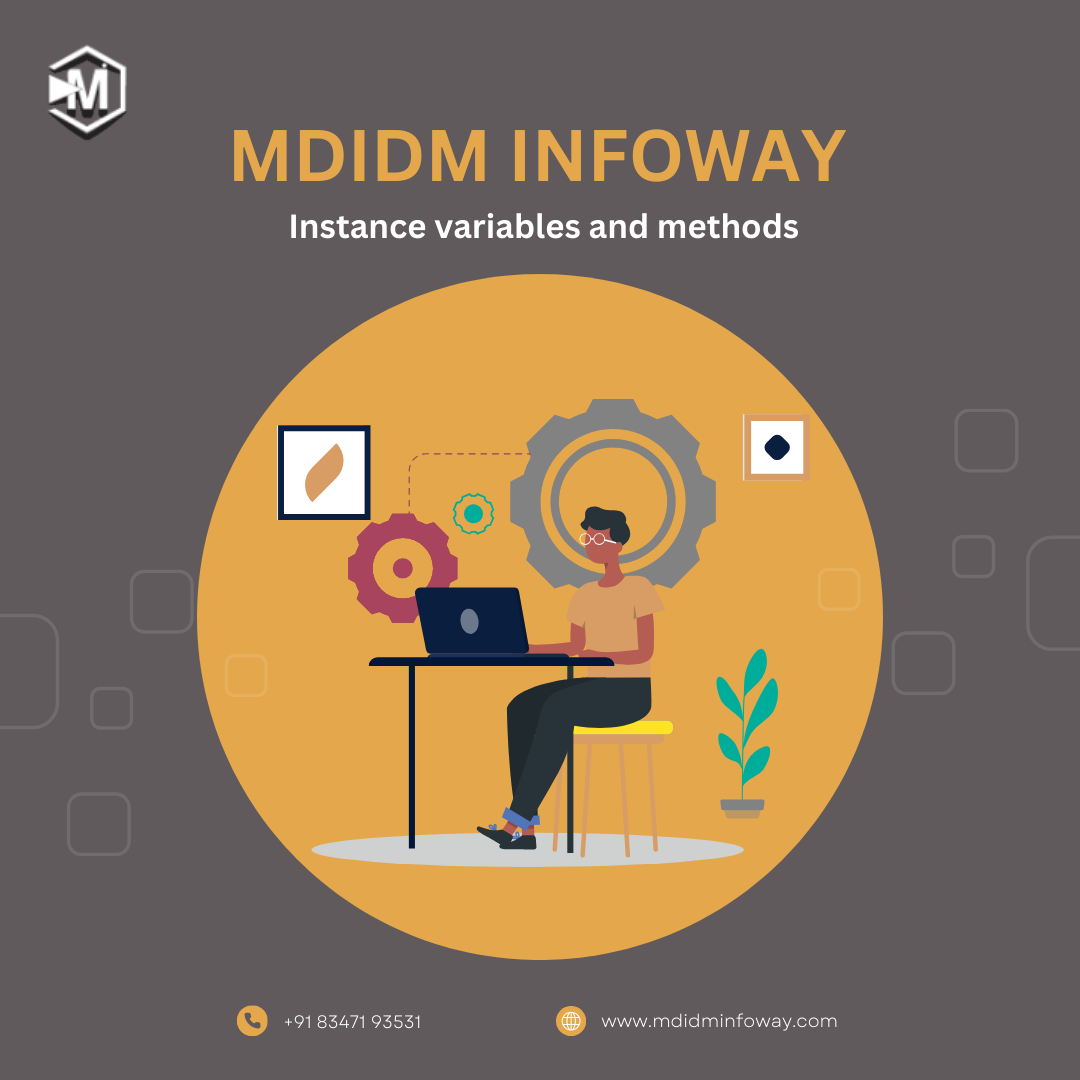
Instance variables and methods
**Instance Variables
Instance variables are attributes that belong to an instance (object) of a class. Each object has its own copy of these variables, any they hold data specific to that object.
**Charactristics of Instance Variables:
•Declared within a class but outside any method.
•Unique for each object(i.e., Changes to one instance variables do not affect others).
•Can be accessed using the self keyword (in python) or this keyword (in java,c++).
•Their values are stored in memory as long as the object exists.
Example in:
class Car:
def __init__(self, brand, color):
self.brand = brand # Instance variable
self.color = color # Instance variable
# Creating objects
car1 = Car("Toyota", "Red")
car2 = Car("Honda", "Blue")
print(car1.brand) # Output: Toyota
print(car2.color) # Output: Blue
2.Instance Methods:
Instance methods are functions desined within a class that operate on instance variables. Thease methods can modify or retrive instance variable values.
Charactristics of Instance Methods:
•Defined inside a class.
•Reuire an instance of the class to be called.
•Can access and modify instance variables.
•Use self to reffrence instance variables.
Example:
class Car:
def __init__(self, brand, color):
self.brand = brand
self.color = color
def display_info(self): # Instance method
return f"Car Brand: {self.brand}, Color: {self.color}"
car1 = Car("Toyota", "Red")
print(car1.display_info()) # Output: Car Brand: Toyota, Color: Red
**Conclusion:
Instance variables and methods are essential in OOP for encapsulating dta and behavior within objects. Proper use of these components ensures modular, reusable, ans well-structured code.