Handling Time Conversions and Time Zones
17 February 2025
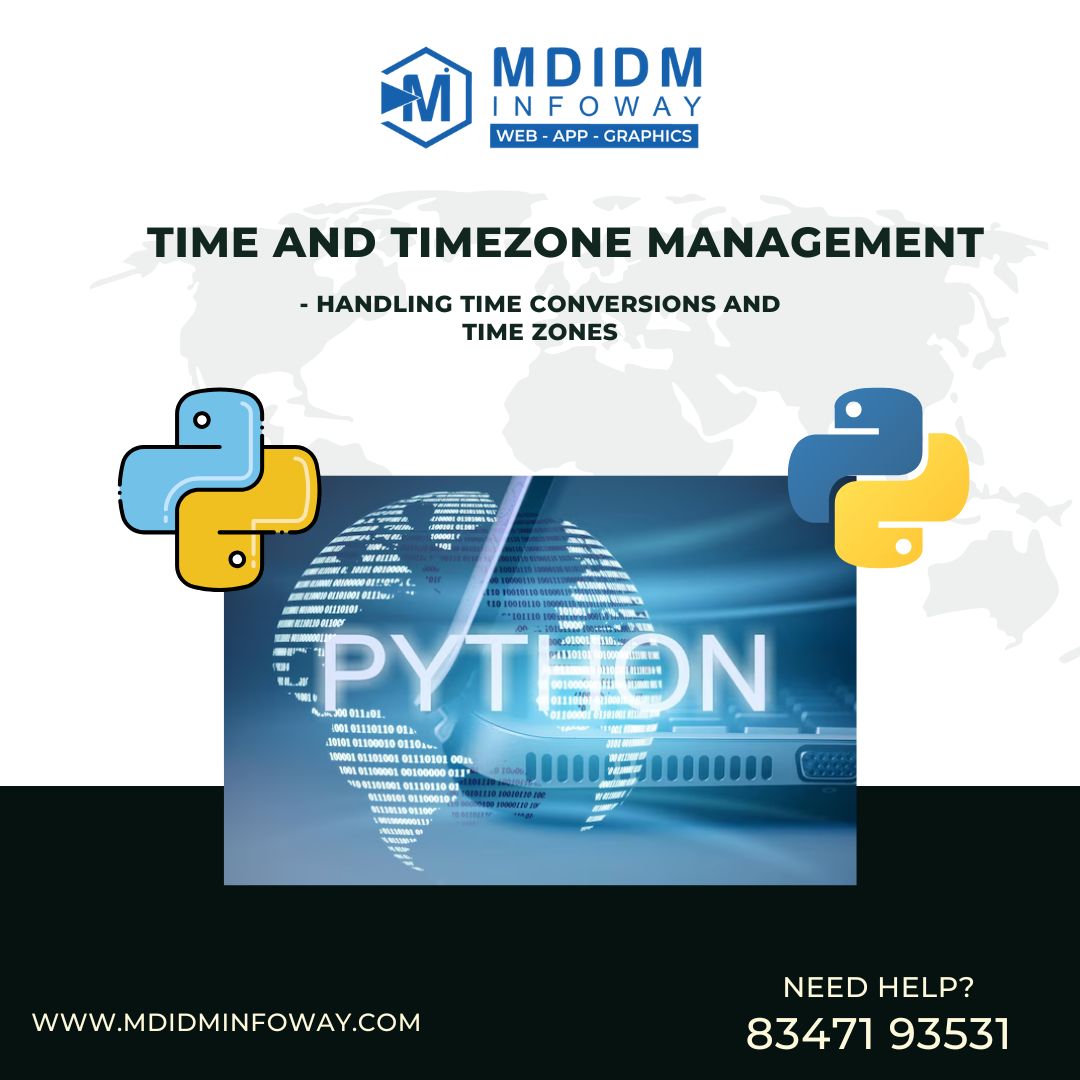
Handling Time Conversions and Time Zones in Python
Working with time zones and conversions is crucial for applications that operate across different regions. Python's datetime and pytz modules provide efficient ways to manage time zone conversions.
1. Importing Required Modules
import datetime
import pytz
2. Getting the Current UTC Time
utc_now = datetime.datetime.utcnow().replace(tzinfo=pytz.utc)
print(utc_now) # Example: 2025-02-15 12:30:45+00:00
3. Converting UTC Time to a Specific Time Zone
local_tz = pytz.timezone("Asia/Kolkata")
local_time = utc_now.astimezone(local_tz)
print(local_time) # Example: 2025-02-15 18:00:00+05:30
4. Converting a Local Time to UTC
local_time = datetime.datetime(2025, 2, 15, 18, 0, 0)
local_time = local_tz.localize(local_time) # Assign time zone
utc_time = local_time.astimezone(pytz.utc)
print(utc_time) # Example: 2025-02-15 12:30:00+00:00
5. Listing Available Time Zones
from pytz import all_timezones
print(all_timezones[:10]) # Displays the first 10 time zones
6. Handling Daylight Saving Time (DST)
Some regions observe DST, which shifts local forward or backward. pytz accounts for these adjustments automatically:
ny_tz = pytz.timezone("America/New_York")
dt = datetime.datetime(2025, 6, 15, 12, 0) # Example date in summer
dt_ny = ny_tz.localize(dt)
print(dt_ny) # Example: 2025-06-15 12:00:00-04:00 (DST applied)
7. Converting Between Time Zones
# Convert New York time to London time
london_tz = pytz.timezone("Europe/London")
dt_london = dt_ny.astimezone(london_tz)
print(dt_london) # Example: 2025-06-15 17:00:00+01:00
Proper time zone handling ensure accurate scheduling, logging, and data synchronization in globally distributed applications.