Function Arguments and Return Values in Python
13 February 2025
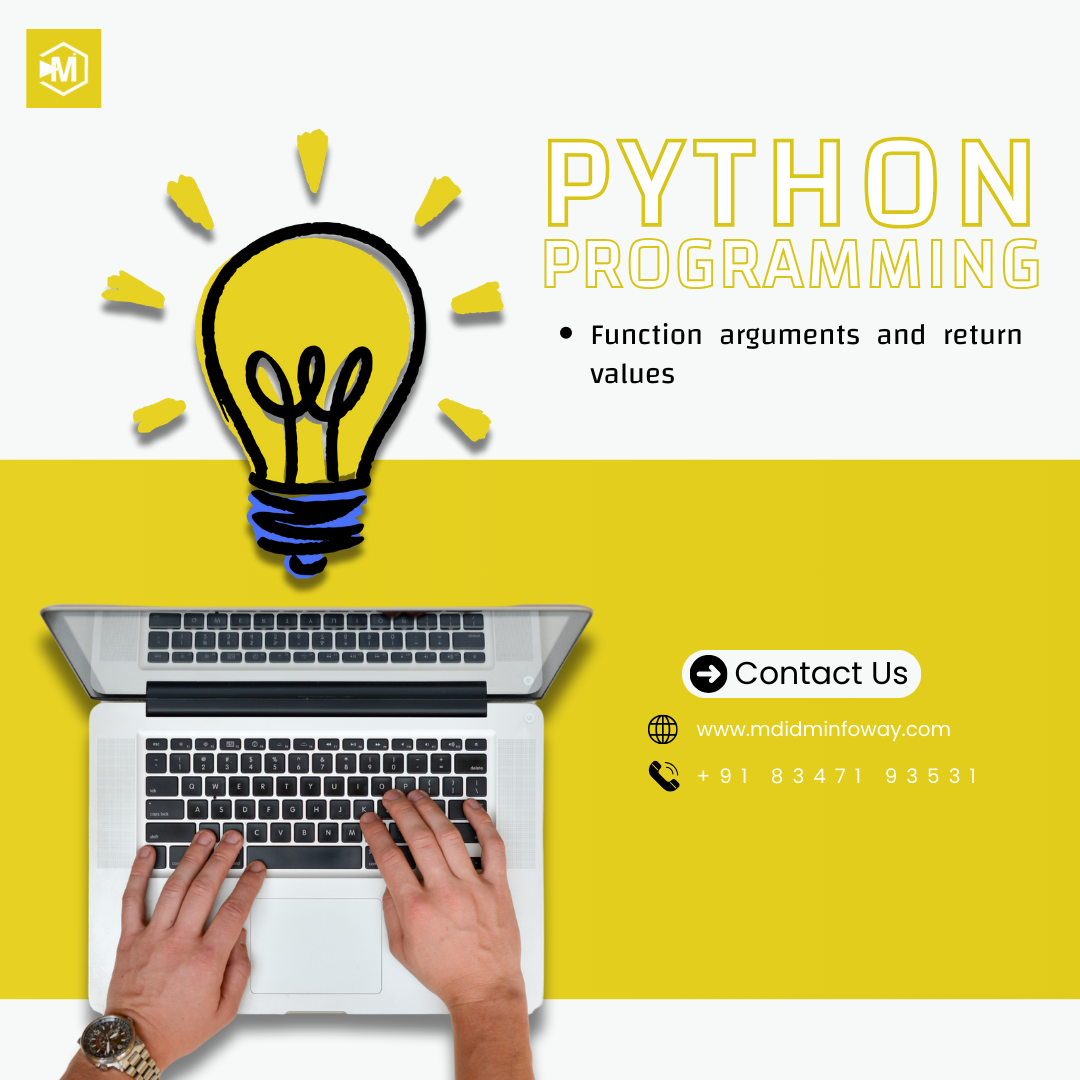
Function Arguments and Return Values in Python
Functions in Python can accept arguments (inputs) and return values (outputs), making them flxible and reusable. Understanding function arguments and return values is essential for writing efficient and modular code.
1. Function Arguments (Parameters)
Function arguments are values passed to a function when it is called. Python supports different types of arguments:
a) Positional Arguments
These are the most common type of arguments, passed in the order they are defined.
Example:
def greet(name, age):
print(f"Hello, {name}! You are {age} years old.")
# Calling the function with positional arguments
greet("Alice", 25)
Output:
Hello, Alice! You are 25 years old.
b) Keyword Arguments
Instead of relying on order, arguments are passed using their names.
Example:
greet(age=30, name="Bob")
Output:
Hello, Bob! You are 30 years old.
c) Default Arguments
If no value is provided, a default value is used.
Example:
def greet(name="Guest"):
print(f"Hello, {name}!")
greet() # Uses default value
greet("Charlie") # Overrides default
Output:
Hello, Guest!
Hello, Charlie!
d) Variable-Length Arguments (*args and **kwargs)
Python allows functions to accept an arbitrary number of arguments.
*args (Non-keyword Arguments)
Allows passing multiple values as a tuple.
def sum_numbers(*args):
return sum(args)
print(sum_numbers(2, 4, 6, 8)) # Accepts multiple numbers
Output:
20
**kwargs (Keyword Arguments)
Allows passing multiple key-value pairs as a dictionary.
def user_info(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
user_info(name="David", age=28, city="New York")
Output:
name: David
age: 28
city: New York
2. Return Values
The return statement is used to send a value back from a functin.
1) Returning a Single Value
def square(num):
return num ** 2
result = square(5)
print("Square:", result)
Output:
Square: 25
b) Returning Multiple Values
Functions can return multiple values as a tuple.
def calculate(a, b):
return a + b, a - b, a * b
sum_val, diff_val, prod_val = calculate(10, 5)
print("Sum:", sum_val, "| Difference:", diff_val, "| Product:", prod_val)
Output:
Sum: 15 | Difference: 5 | Product: 50
Key Taleaways:
- Arguments provide flexibility by allowing different types of inputs.
- Return values make functions useful by sending back results.
- Using *args and **kwargs allows handling dynamic numbers of inputs.
Mastering function arguments and return values helps in writing modular, reusable, and scalable code.