Python Programming Core Programming Concepts
13 February 2025
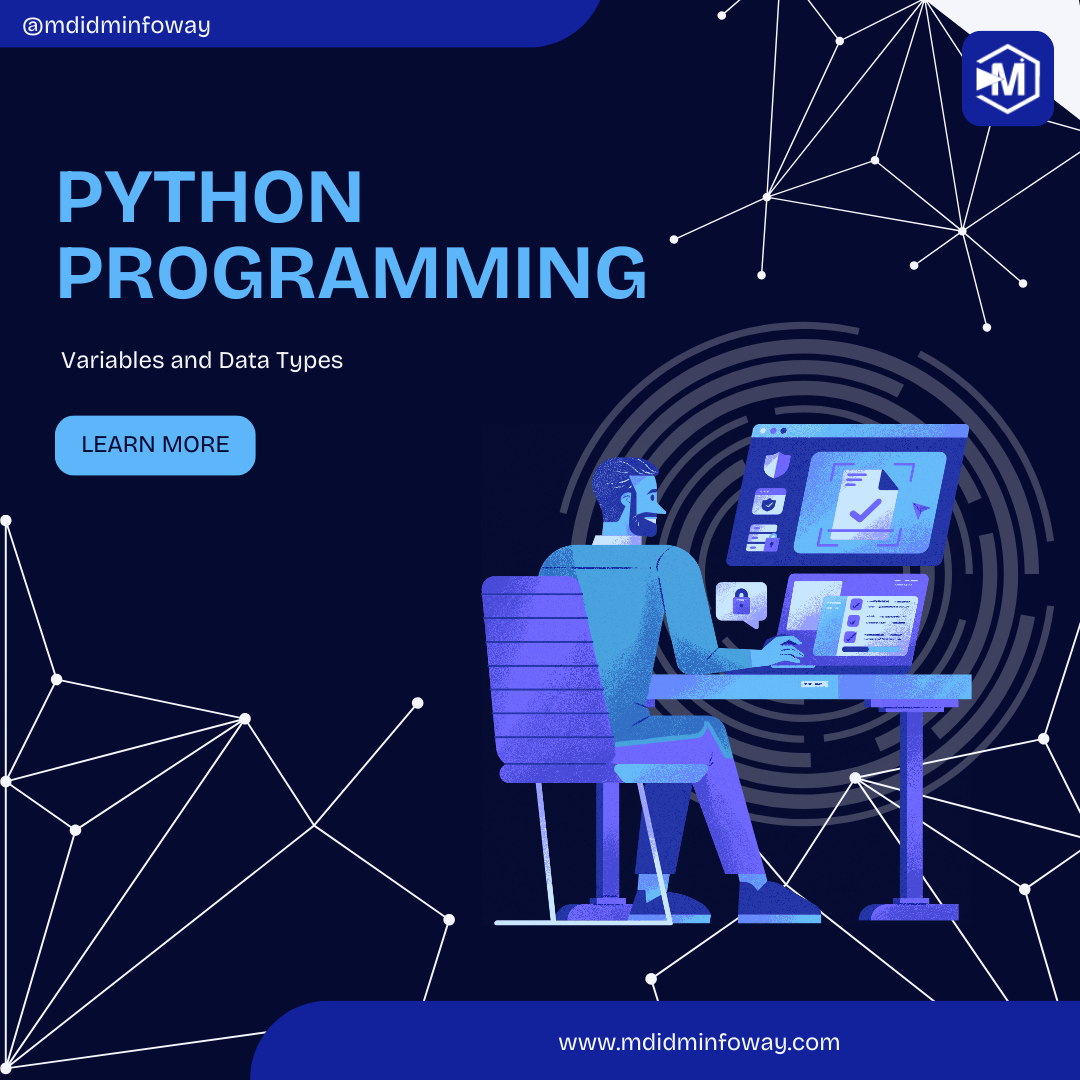
Python Programming : Core Programming Concepts
Python programming is built on several Core programming concept that form the foundation of writing efficient and effective code.
1.Variables and Data Types
•Variables store data values.
•Data types define the kind of data a variable can hold.
•Common data types:
•int(integer)
•float(decimal numbers)
•str(string/text)
•bool(True/False)
•list(ordered, mutable collection0
•tuple(ordered, immutable collection)
•dict(key-value pairs)
•set(unordered unique iteams)
Example :
age = 25 #int
height = 5.9 #float
name = "Alice" #str
is_student = True #bool
2.Operators
•Used to perfom operations on variables and values.
•Types of operators :
•Arithmetic operators (+, -, , /, %, *, //)
•Comparison operators (==, !=, >, <, >=, <=)
•Logical operators (and, or, not)
•Bitwise operators (&, |, ~, <<, >>)
•Assignment operators (=, +=, -=, *=, /=, %=)
•Membership operators (in, not in)
•Identity operators (is, is not)
Example :
x,y = 10, 5
print(x+y) #15
print(x>y and y<20) #True
3.Control Flow Statements
•Conditional Statements(if, elif, else): Control decision-making in a program.
•Loops (for, while): Execute repetitive tasks.
Example :
1. num = 10
if num>0:
print("positive number")
elif num<0:
print("Negative number")
else:
print("Zero")
2. for i in range(5):
print(i) # Prints 0 to 4
count = 0
while count < 3:
print(count)
count += 1
4.Functions
•Function allows code reuse and modularity.
•Built-in Funtiona: print(), len(), input(), type(), etc.
•User-defined Functions:
Example:
def greet(name):
return f"Hello, {name}!"
Print(greet("Alice"))
5.Data Structures
•Lists: Ordered, mutable collections.
•Truples: Ordered, immutable collections.
•Dictionaries: Key-value pairs.
•Sets: Unordered, unique values.
Example:
my_list=[1,2,3]
my_tuple=(4,5,6)
my_dict={"name" : "Alice", "age" : 25}
my_set = {1,2,3,3} #{1,2,3} (duplicates removed)
6.Exception Handling
•Used to handle errors and prevent program crashes.
Example:
try:
x=10/0
except ZeroDivisionError:
print("Cannot divide by zero!")
Finally:
print("Execution complete.")
7.Object-Oriented Programming (OOP)
•Classes and Objects: Code is structured using objects.
class person:
def_init_(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f'My name is {self.name} and I am {self.age} years old."
person1=person("Alice", 25)
print(person1.introduce())
Thease core programming concepts from the backbone of pythn programming and mastering them will elp you write efficient and scalable programs.