Python Lists Creation Manipulation Slicing and Methods
13 February 2025
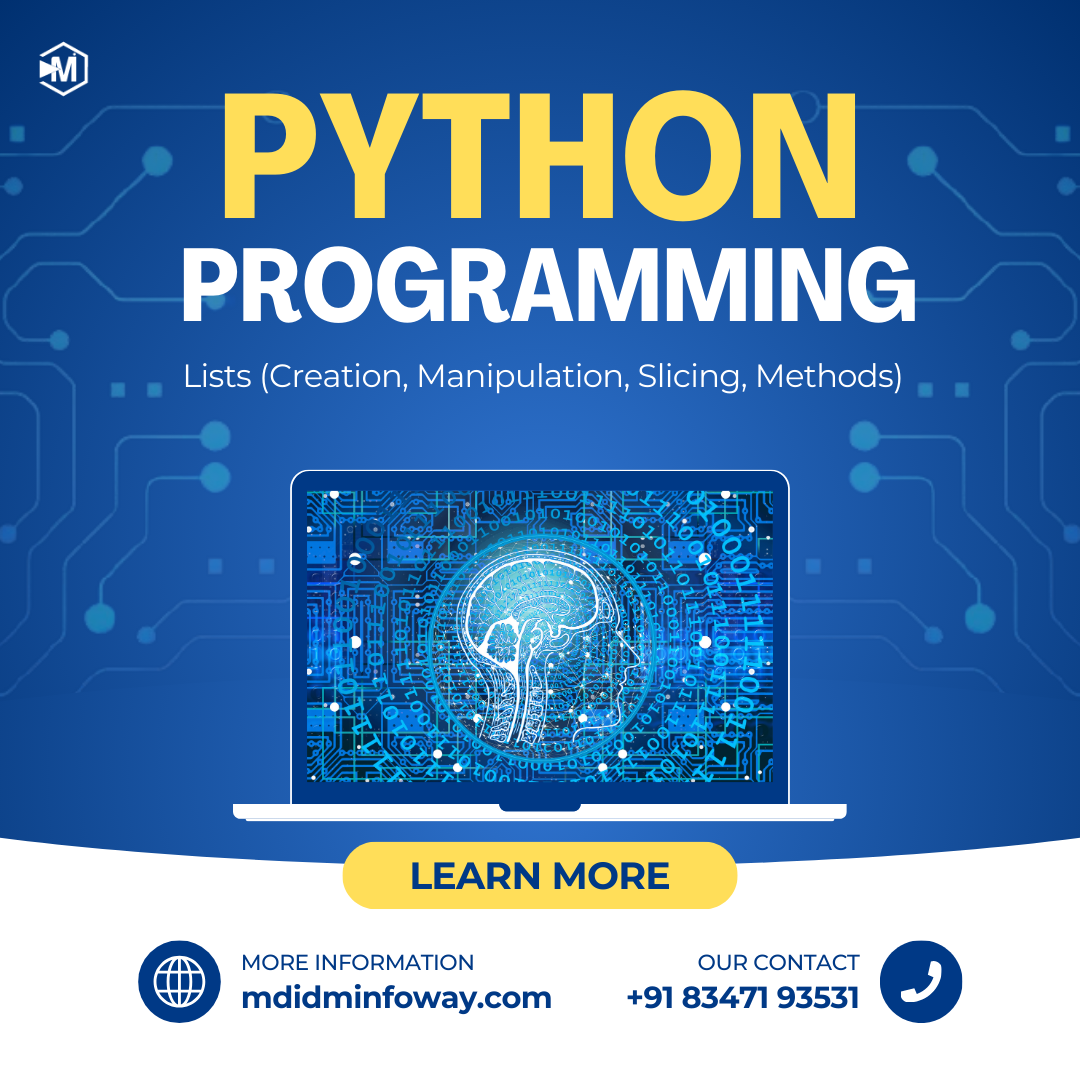
Python Lists: Creation, Manipulation, Slicing, and Methods
A list in Python is an ordered, mutable, and heterogeneous collection of elements. Lists allow storing multiple items in a single variable and support various opertions for easy manipulation.
1. Creating a List:
Lists are defined using square breackets [], and elements are separated by commas.
Examples:
# Creating lists
empty_list = [] # An empty list
numbers = [1, 2, 3, 4, 5] # List of integers
fruits = ["apple", "banana", "cherry"] # List of strings
mixed = [10, "hello", 3.14, True] # Mixed data types
# Printing lists
print(numbers) # Output: [1, 2, 3, 4, 5]
print(fruits) # Output: ['apple', 'banana', 'cherry']
2. Accessing and Modifying List Elements
a) Accessing Elements(Indexing and Negative Indexing)
fruits = ["apple", "banana", "cherry"]
# Access by index (0-based)
print(fruits[0]) # Output: apple
print(fruits[2]) # Output: cherry
# Negative indexing (last element first)
print(fruits[-1]) # Output: cherry
b) Modifying Elements
fruits[1] = "blueberry" # Changing 'banana' to 'blueberry'
print(fruits) # Output: ['apple', 'blueberry', 'cherry']
3. Slicing Lists:
Slicing extracts a portion of a list using the syntax
list[start:stop:step].
numbers = [10, 20, 30, 40, 50, 60, 70]
print(numbers[1:4]) # Output: [20, 30, 40] (Index 1 to 3)
print(numbers[:3]) # Output: [10, 20, 30] (Start from index 0)
print(numbers[3:]) # Output: [40, 50, 60, 70] (Till end)
print(numbers[::2]) # Output: [10, 30, 50, 70] (Step of 2)
print(numbers[::-1]) # Output: [70, 60, 50, 40, 30, 20, 10] (Reverse list)
4. Common List Methods
Python provides built-in methods to manipulate lists easily.
a) Adding Elements
fruits = ["apple", "banana"]
fruits.append("cherry") # Adds 'cherry' at the end
print(fruits) # Output: ['apple', 'banana', 'cherry']
fruits.insert(1, "orange") # Inserts 'orange' at index 1
print(fruits) # Output: ['apple', 'orange', 'banana', 'cherry']
b) Removing Elements
fruits.remove("banana") # Removes 'banana'
print(fruits) # Output: ['apple', 'orange', 'cherry']
last_fruit = fruits.pop() # Removes and returns the last element
print(last_fruit) # Output: cherry
print(fruits) # Output: ['apple', 'orange']
fruits.clear() # Removes all elements
print(fruits) # Output: []
c) Searching Elements
numbers = [10, 20, 30, 40]
print(numbers.index(30)) # Output: 2 (Index of 30)
print(20 in numbers) # Output: True (Checks if 20 exists)
d) Sorting and Reversing
nums = [4, 1, 8, 3]
nums.sort() # Sorts in ascending order
print(nums) # Output: [1, 3, 4, 8]
nums.reverse() # Reverses the list
print(nums) # Output: [8, 4, 3, 1]
e) Copying a List
original = [1, 2, 3]
copy_list = original.copy()
print(copy_list) # Output: [1, 2, 3]
5. List Comprehension (Efficient Way to Create Lists)
List comprehension provides a concise way to create lists dynamically.
# Creating a list of squares
squares = [x ** 2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
# Filtering even numbers
evens = [x for x in range(10) if x % 2 == 0]
print(evens) # Output: [0, 2, 4, 6, 8]
Key Takeaways
- Lists are mutable and allow heterogeneous data.
- Indexing and slicing help access manipulate data efficiently.
- List methods simplify data operations like adding, removing, and sorting elements.
- List comprehensions offer a powerful way to generate lists dynamically.
Lists are a fundamental data structure in Python, making data storage and manipulation simple and efficient!