Mastering Flutter From Basics to Pro
1 February 2025
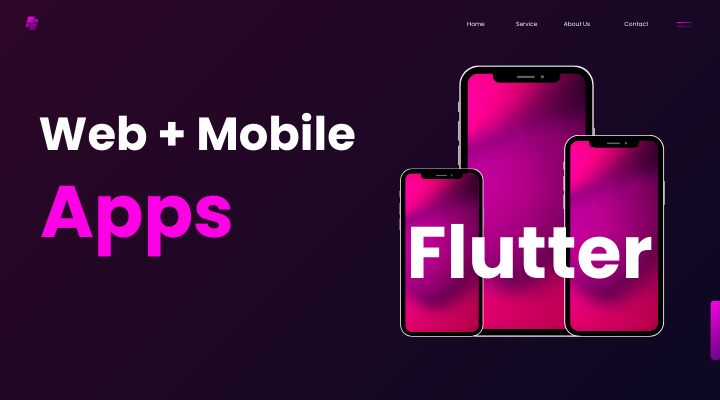
Mastering Flutter: From Basics to Pro
Flutter has emerged as one of the most powerful and versatile frameworks for cross-platform app development. Whether you're a beginner just getting started or a developer looking to level up your skills, this guide will help you master Flutter from the ground up.
Why Choose Flutter?
Flutter, developed by Google, is an open-source UI toolkit that allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. It offers:
- Fast Development: With the hot reload feature, developers can see changes instantly.
- Expressive UI: Flutter provides customizable widgets for a smooth and responsive user experience.
- Single Codebase: Write once, deploy on Android, iOS, web, and more.
- Strong Community Support: A vast community and extensive documentation make learning easier.
Getting Started with Flutter
Before diving into advanced topics, it's essential to set up your development environment:
1. Install Flutter SDK: Download from the official Flutter website.
2. Set Up an Editor: VS Code and Android Studio are popular choices.
3. Create Your First App:
flutter create my_first_app
cd my_first_app
flutter run
Understanding Flutter Architecture
Flutter’s architecture is based on three primary layers:
- Framework Layer: Provides UI elements like widgets and animations.
- Engine Layer: Handles rendering, text layout, and graphics.
- Embedder Layer: Integrates with platforms like Android and iOS.
Moving from Basics to Advanced Topics
1. Mastering Widgets
Flutter revolves around widgets. Understanding their types helps in creating efficient UI designs:
Stateless Widgets: Do not change once built (e.g., Text, Container).
Stateful Widgets: Maintain state and update dynamically (e.g., TextField, Checkbox).
2. State Management
State management is crucial for building scalable applications. Some popular approaches include:
setState(): Best for simple applications.
Provider: Recommended for scalable apps.
Riverpod, BLoC, Redux: Used in large-scale applications.
3. Handling Navigation
Flutter provides multiple navigation techniques:
Navigator.push(): Simple navigation between screens.
Named Routes: Organized way of handling navigation.
GoRouter: An advanced routing package for better control.
4. Working with APIs
Fetching data from the internet is common in modern apps. Use the http package to make API calls:
import 'package:http/http.dart' as http;
Future fetchData() async {
final response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200) {
return response.body;
} else {
throw Exception('Failed to load data');
}
}
5. Optimizing Performance
To make your Flutter app run efficiently:
Use const widgets whenever possible.
Minimize widget rebuilds.
Optimize images and assets.
Implement lazy loading techniques.
Becoming a Pro Flutter Developer
To transition from a beginner to an expert, consider:
Building Real Projects: Practice by creating apps like To-Do lists, weather apps, or e-commerce platforms.
Exploring Flutter Packages: Utilize plugins like firebase_auth, sqflite, and flutter_local_notifications.
Contributing to Open Source: Join Flutter repositories on GitHub.
Keeping Up with Updates: Follow Flutter's official blog and community forums.
Conclusion
Mastering Flutter takes practice, patience, and continuous learning. With a solid understanding of widgets, state management, navigation, and API integration, you can develop high-performance cross-platform apps. Keep experimenting and building projects to refine your skills further. Happy coding!