Loop Control Statements in Python
13 February 2025
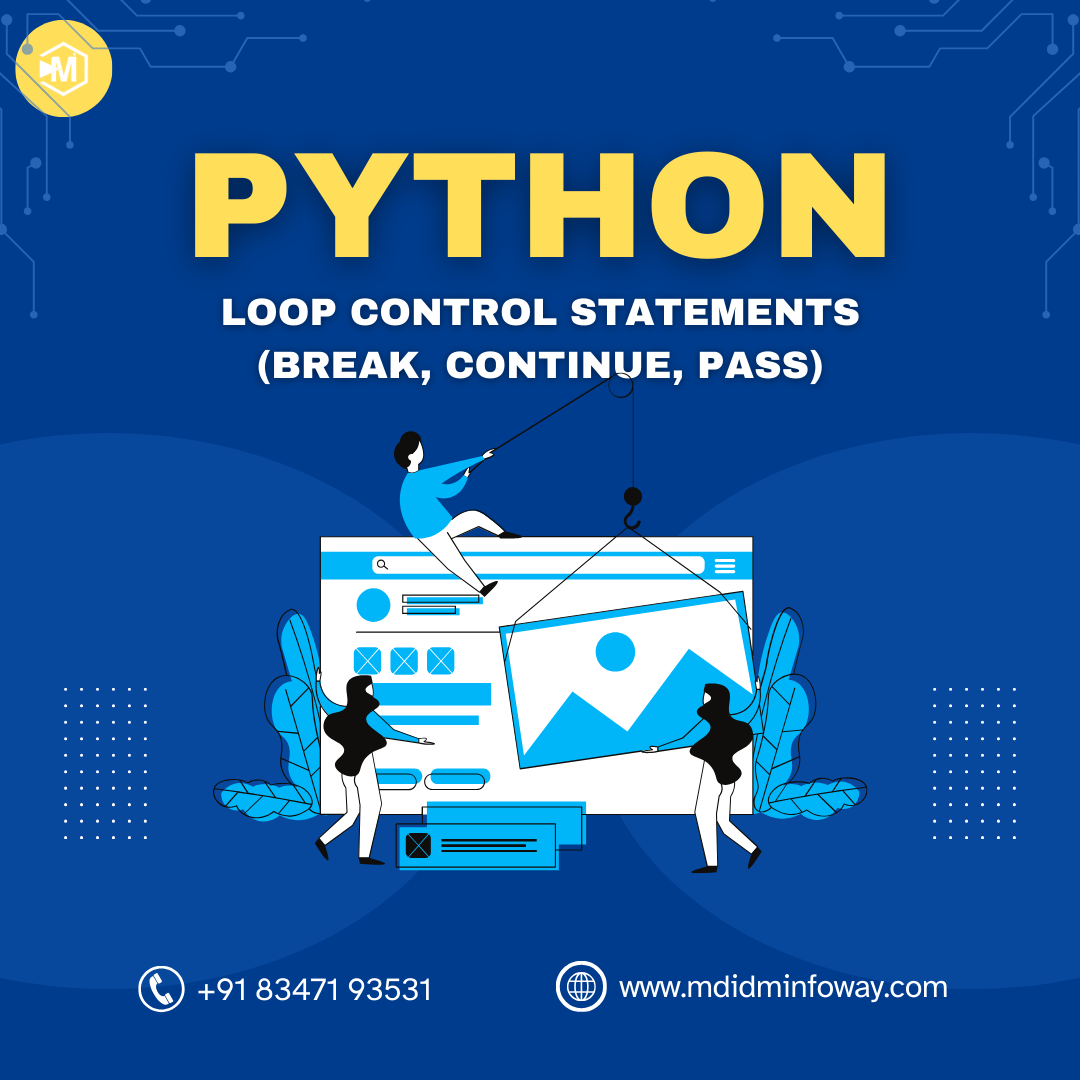
Loop Control Statements in Python
Loop control statements alter the normal flow of a loop by stopping or skipping iterations based on specific conditions. Python provides three main loop control statements:
1. break - Terminates the loop entirely.
2. Continue - Skips the current iterations and moves to the next one.
3. pass - Acts as a placeholder, allowing the loop to run without performing any action.
1. break Statement:
The break statement is used to exit a loop immediately when a condition is met.
Example: Breaking a Loop When a Specific Value is Found
for number in range(1, 10):
if number == 5:
break # Loop terminates when number is 5
print(number)
Output:
1
2
3
4
2. Continue Statement
The continue statement skips the current iteration and moves to the next one, without exiting the loop.
Example: Skipping Even Numbers
for number in range(1, 6):
if number % 2 == 0:
continue # Skips even numbers
print(number)
Output:
1
3
5
3. pass Statement:
The pass statement does nothing and is used as a placeholder when a block of code is syntactically required but not yet implemented.
Example: Using pass in a Loopfor number in range(1, 6):
if number == 3:
pass # Placeholder for future implementation
else:
print(number)
Output:
1
2
4
5
Key Differences Between Loop Control Statements
Statement: break
Effect: Exits the loop immediately.
Statement: continue
Effect: Skips the current iteration and moves to the next one.
Statement: pass
Effect: Does nothing, used as a placeholder.
These loop control statements enhance flexibility in loop execution, making code more efficient and readable.