Encapsulation Private Protected Public members
18 February 2025
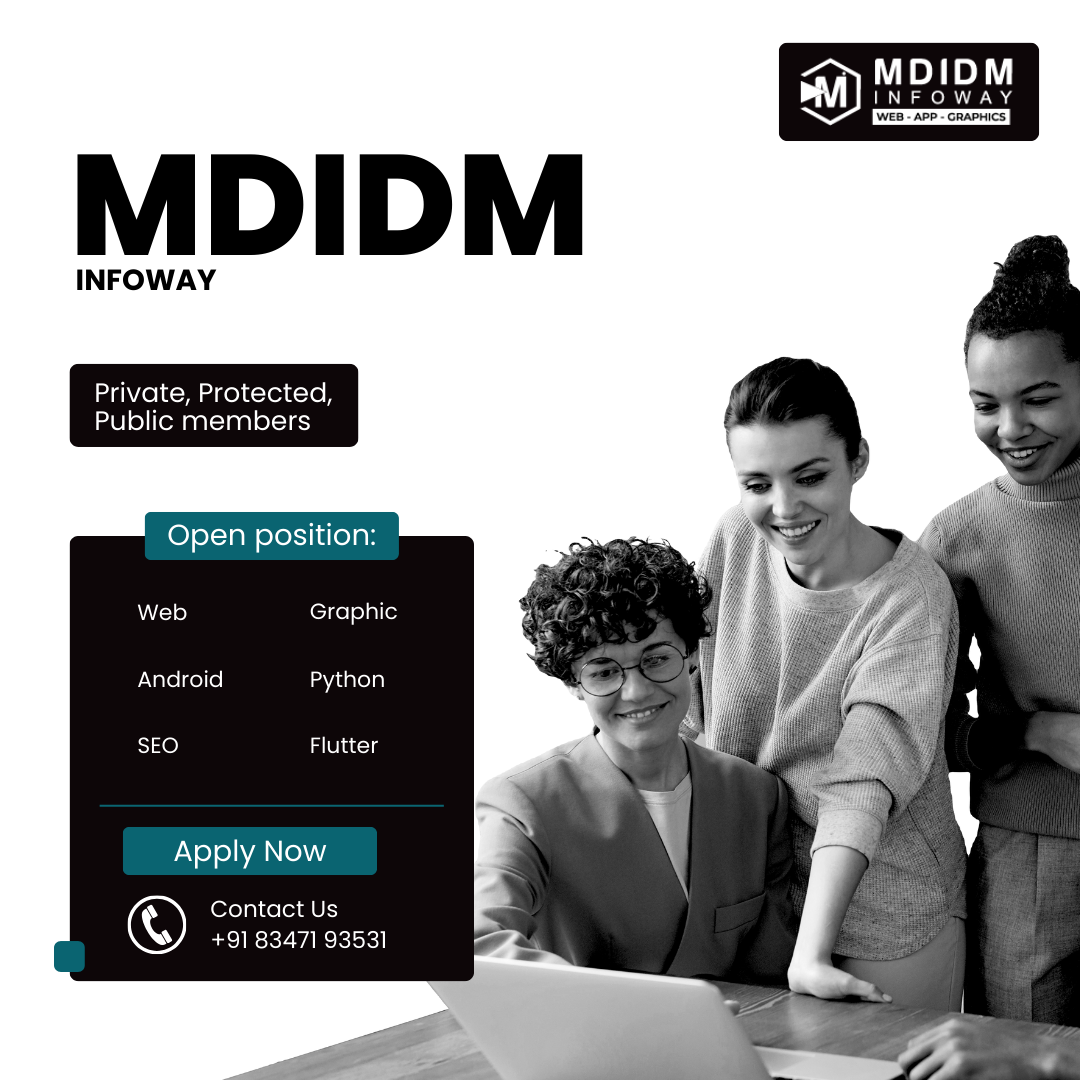
- Encapsulation: Private, Protected, Public members
**Encapsulation:
Encapsulation is the process of wrapping data(variables) and code (methods) into a single unit(class) and restricting direct access to certain details. It helps in sata security, modularity and maintainability.
**Access Modifiers in Encapsulation
Encapsulation is implemented using access modifiers that define the visibility of class members.
Private(private):
•members are accessible only within the same class.
•Ensures data security by preventing direct modification from outside the class.
Example :
class Account {
private double balance = 1000.0;
public double getBalance() {
return balance;
}
public void setBalance(double amount) {
if (amount > 0) {
balance = amount;
}
}
}
Protected(protected)
•Members are accessible within the same package and by subclasses (even if they are in diffrent packages).
•Useful in inheritance when child classes need access to certain properties of parents class.
Example:
class Person {
protected String name;
}
class Employee extends Person {
void showName() {
System.out.println("Employee Name: " + name);
}
}
Public (public)
Members are accessible from anywhere in the program.
Used for methods or variables that need to be widely available.
Example:
class Car {
public String brand = "Toyota";
}
class Main {
public static void main(String[] args) {
Car myCar = new Car();
System.out.println(myCar.brand); // Accessible from anywhere
}
}
Encapsulation enhances security, data hiding and code organization by controlling access to class members.